Boost Your Code Quality with These Easy-to-Implement Principles
Learn how to boost code clarity and efficiency with simple, effective practices you can apply today.
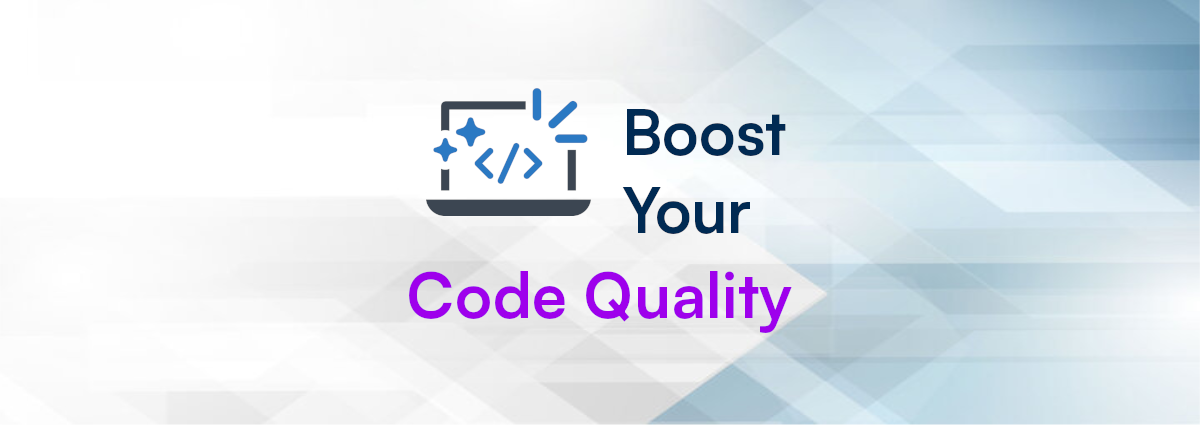
October has arrived, bringing a sense of renewal as autumn sets in. This season often takes me back to my university days, prompting reflections on my relatively brief journey in software development. During my studies, I was fixated on solving the most challenging problems, often overlooking the process by which I reached the solutions. However, after starting my professional career, I quickly came to the stark realization that simply solving coding problems wasn't enough. The importance of writing code correctly, in some cases, even surpassed that of finding a solution.
In this article, I would like to share some straightforward principles that, when effectively incorporated into our day-to-day tasks, can significantly elevate the quality of our code.
Readability
The importance of readability cannot be overstated. A valuable piece of advice to remember is "code is read more often than it is written". This is true because writing code is an iterative process, with each iteration we build on the code produced from the last, adding features, fixing bugs etc. In order to do this you need to be able to read the existing code. Therefore, as much effort should be invested in enhancing code readability as is applied in creating a functioning solution.
Naming
Effective coding begins with appropriate naming of classes, functions, and variables. We tend to name things for their purpose, and the same rule applies in programming. Object names should reflect their role in code rather than their nature. Consider this example:
temperature_readings = [16, 21, 19, 23, 18]
# poor naming
result = max(temperature_readings)
# better naming
max_temperature = max(temperature_readings)
It's important to follow the naming convention of the programming language used (snake_case, camelCase, etc.). It's also recommended to avoid abbreviations unless they're widely recognized, such as those found on Wikipedia. This could lead to longer variable names but increases readability.
Formatting
Proper formatting keeps code readable and helps teams work together smoothly. Consistency speeds up understanding and navigation. In Python,PEP 8 sets the style, while tools like black
apply it automatically, shaping up code fast so developers can focus on the bigger picture.
Comments
Comments should be used judiciously. Well-written code often serves as its own documentation. Overuse of comments can lead to unnecessary duplication, as illustrated here:
# Convert June temperatures to CSV
june_temperatures.to_csv('june_temperatures.csv')
That said, comments have their place, particularly for explaining complex logic, workarounds, or specific configuration choices. These comments can save other developers time in understanding the purpose or context of such code sections.
Programming principles
DRY - DON’T REPEAT YOURSELF
Avoid duplicating logic. If you find yourself copying and pasting code, consider refactoring into a function or module. Reducing repetition keeps the code base concise and makes it easier to implement changes.
# DUPLICATED CODE
# Rectangle 1
length1 = 5
width1 = 10
area1 = length1 * width1
# Rectangle 2
length2 = 7
width2 = 3
area2 = length2 * width2
# DRY CODE
def calculate_rectangle_area(length, width):
return length * width
# Usage
area1 = calculate_rectangle_area(5, 10)
area2 = calculate_rectangle_area(7, 3)
KISS - KEEP IT STUPID SIMPLE
Strive for simplicity. Don't use a complex algorithm when a straightforward solution suffices. Complex code is hard to read(again with the readability it’s very important), debug, and maintain.
def is_even(num):
return True if num % 2 == 0 else False
def is_even(num):
return num % 2 == 0
YAGNI YOU AREN’T GONNA NEED IT
Avoid creating functions that are not essential. Focus on what is really necessary, keeping the code simple and direct. The YAGNI practice is important for two main reasons:
- Time Savings: You stop spending time developing functions that you may never use.
- Cleaner Code: Your code becomes clearer and free of assumptions that often turn out to be wrong.
def calculate_metrics(data, advanced=False):
if advanced: # Premature implementation of an unused feature
perform_complex_analysis(data)
return simple_analysis(data)
def calculate_metrics(data):
return simple_analysis(data) # Implement only what's needed now
Data structures
Choosing the appropriate data structure can dramatically improve the readability and simplicity of your code. The right data structure can reduce complexity and increase the efficiency of your algorithms. Here is an example:
# Using a list of tuples to represent a user's properties
user_data = [('name', 'Alice'), ('age', 30), ('active', True)]
# Accessing data requires iterating through the list or having
# prior knowledge of index
user_age = next(value for key, value in user_data if key == 'age')
# Using a dictionary to represent a user's properties
user_data = {'name': 'Alice', 'age': 30, 'active': True}
user_age = user_data['age']
Learning
- practice
- Practicing coding regularly is essential for retaining knowledge and improving skills. It's similar to learning a musical instrument; the more you practice, the more fluent you become.
- Building small projects, solving coding challenges, and contributing to open-source projects are great ways to apply what you've learned.
- Consistent practice helps in reinforcing concepts and developing problem-solving skills.
- Read code
- Reading the code written by others can be as advantageous as writing your own. It exposes you to different coding styles, paradigms, and best practices.
- By reading well-written code, you can learn how to structure your projects effectively and how certain problems can be approached.
- Use modern tools like chatgpt, github co-pilot carefully:
- Tools like ChatGPT and GitHub Copilot tools should be used carefully to ensure that they do not become a crutch. Relying on them without understanding the underlying code can lead to a superficial grasp of programming concepts.
- Avoid Uncritical Copying:
- Never copy code without trying to understand it. This can lead toproblems when the code doesn't work as expected or needs to be modified, and you don't understand how to fix it.
- Write It to Learn It:
- If you need to use a piece of code that you did not write yourself(e.g., a solution from Stack Overflow or a snippet suggested by GitHubCopilot), don't just copy and paste; instead, type it out line by line.
To sum it up, writing clean code is a balanced act of adhering to good practices, being mindful of readability, selecting the right tools for the challenge at hand. I always try to remember that enhancing one’s coding skills is a gradual process. It requires practice, patience, and consistent learning.
I would also like to thank my first mentor francois granade. His patience and guidance during the first 2 years of my software development journey instilled a deep understanding and appreciation for clean coding, the principles of which I've shared some with you in this article.
Happy coding!